The Spring Framework is an open source application framework and Inversion of Control container for the Java platform. The core features of the Spring Framework can be used by any Java application, but there are extensions for building web applications on top of the Java EE platform. Although the Spring Framework does not impose any specific programming model, it has become popular in the Java community as an alternative to, replacement for, or even addition to the Enterprise JavaBean (EJB) model.
Modules
The Spring Framework comprises several modules that provide a range of services:
Modules
The Spring Framework comprises several modules that provide a range of services:
- Inversion of Control container: configuration of application components and lifecycle management of Java objects, done mainly via Dependency Injection
- Aspect-oriented programming: enables implementation of cross-cutting routines
- Data access: working with relational database management systems on the Java platform using JDBC and object-relational mapping tools and with NoSQL databases
- Transaction management: unifies several transaction management APIs and coordinates transactions for Java objects
- Model-view-controller: an HTTP- and servlet-based framework providing hooks for extension and customization for web applications and RESTful web services.
- Remote Access framework: configurative RPC-style export and import of Java objects over networks supporting RMI(Java Remote Method Invocation), CORBA and HTTP-based protocols including web services (SOAP-Simple Object Access Protocol)
- Convention-over-configuration: a rapid application development solution for Spring-based enterprise applications is offered in the Spring Roo module
- Batch processing: a framework for high-volume processing featuring reusable functions including logging/tracing, transaction management, job processing statistics, job restart, skip, and resource management
- Authentication and authorization: configurable security processes that support a range of standards, protocols, tools and practices via the Spring Security sub-project (formerly Acegi Security System for Spring).
- Remote Management: configurative exposure and management of Java objects for local or remote configuration via JMX (Java Management Extensions)
- Messaging: configurative registration of message listener objects for transparent message-consumption from message queues via JMS, improvement of message sending over standard JMS (Java Message Service) APIs
- Testing: support classes for writing unit tests and integration tests
Advantages of Spring Framework :
- Spring is Lightweight container
- No App Server Dependent – like EJB JNDI (Enterprise Java Beans - Java Naming and Directory Interface) Calls
- Objects are created Lazily , Singleton - configuration
- Components can added Declaratively
- Initialization of properties is easy – no need to read from properties file
- Declarative transaction, security and logging service - AOP(Aspect-Oriented Programming)
- application code is much easier to unit test
- With a Dependency Injection approach, dependencies are explicit, and evident in constructor or JavaBean properties
- Spring's configuration management services can be used in any architectural layer, in whatever runtime environment.
- Spring can effectively organize your middle tier objects
- Not required special deployment steps
The Spring framework
The Spring framework is a layered architecture consisting of seven well-defined modules. The Spring modules are built on top of the core container, which defines how beans are created, configured, and managed, as shown in Figure 1.
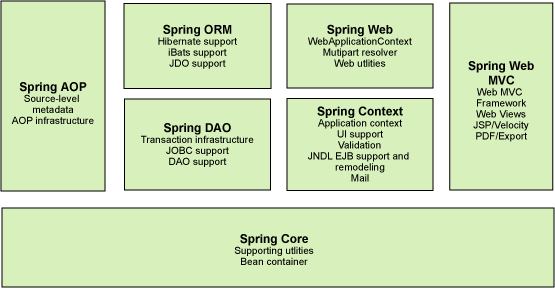
Each of the modules (or components) that comprise the Spring framework can stand on its own or be implemented jointly with one or more of the others. The functionality of each component is as follows:
The core container: The core container provides the essential functionality of the Spring framework. A primary component of the core container is the BeanFactory, an implementation of the Factory pattern. The BeanFactory applies the Inversion of Control (IOC) pattern to separate an application's configuration and dependency specification from the actual application code.
Spring context: The Spring context is a configuration file that provides context information to the Spring framework. The Spring context includes enterprise services such as JNDI, EJB, e-mail, internalization, validation, and scheduling functionality.
The Spring framework is a layered architecture consisting of seven well-defined modules. The Spring modules are built on top of the core container, which defines how beans are created, configured, and managed, as shown in Figure 1.
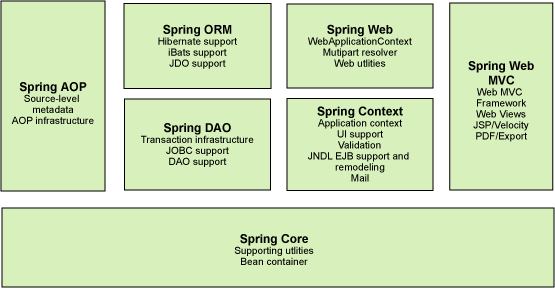
Each of the modules (or components) that comprise the Spring framework can stand on its own or be implemented jointly with one or more of the others. The functionality of each component is as follows:
The core container: The core container provides the essential functionality of the Spring framework. A primary component of the core container is the BeanFactory, an implementation of the Factory pattern. The BeanFactory applies the Inversion of Control (IOC) pattern to separate an application's configuration and dependency specification from the actual application code.
Spring context: The Spring context is a configuration file that provides context information to the Spring framework. The Spring context includes enterprise services such as JNDI, EJB, e-mail, internalization, validation, and scheduling functionality.
Spring AOP: The Spring AOP module integrates aspect-oriented programming functionality directly into the Spring framework, through its configuration management feature. As a result you can easily AOP-enable any object managed by the Spring framework. The Spring AOP module provides transaction management services for objects in any Spring-based application. With Spring AOP you can incorporate declarative transaction management into your applications without relying on EJB components.
Spring DAO: The Spring JDBC DAO abstraction layer offers a meaningful exception hierarchy for managing the exception handling and error messages thrown by different database vendors. The exception hierarchy simplifies error handling and greatly reduces the amount of exception code you need to write, such as opening and closing connections. Spring DAO's JDBC-oriented exceptions comply to its generic DAO exception hierarchy.
Spring ORM: The Spring framework plugs into several ORM frameworks to provide its Object Relational tool, including JDO (Java Data Objects), Hibernate, and iBatis SQL Maps. All of these comply to Spring's generic transaction and DAO exception hierarchies.
Spring Web module: The Web context module builds on top of the application context module, providing contexts for Web-based applications. As a result, the Spring framework supports integration with Jakarta Struts. The Web module also eases the tasks of handling multi-part requests and binding request parameters to domain objects.
Spring MVC framework: The Model-View-Controller (MVC) framework is a full-featured MVC implementation for building Web applications. The MVC framework is highly configurable via strategy interfaces and accommodates numerous view technologies including JSP, Velocity, Tiles, iText, and POI.
Spring framework functionality can be used in any J2EE server and most of it also is adaptable to non-managed environments. A central focus of Spring is to allow for reusable business and data-access objects that are not tied to specific J2EE services. Such objects can be reused across J2EE environments (Web or EJB), standalone applications, test environments, and so on, without any hassle.
The benefits Spring can bring to a project:
- Spring can effectively organize your middle tier objects, whether or not you choose to use EJB. Spring takes care of plumbing that would be left up to you if you use only Struts or other frameworks geared to particular J2EE APIs. And while it is perhaps most valuable in the middle tier, Spring's configuration management services can be used in any architectural layer, in whatever runtime environment.
- Spring can eliminate the proliferation of Singletons seen on many projects. In my experience, this is a major problem, reducing testability and object orientation.
- Spring can eliminate the need to use a variety of custom properties file formats, by handling configuration in a consistent way throughout applications and projects. Ever wondered what magic property keys or system properties a particular class looks for, and had to read the Javadoc or even source code? With Spring you simply look at the class's JavaBean properties or constructor arguments. The use of Inversion of Control and Dependency Injection (discussed below) helps achieve this simplification.
- Spring can facilitate good programming practice by reducing the cost of programming to interfaces, rather than classes, almost to zero.
- Spring is designed so that applications built with it depend on as few of its APIs as possible. Most business objects in Spring applications have no dependency on Spring.
- Applications built using Spring are very easy to unit test.
- Spring can make the use of EJB an implementation choice, rather than the determinant of application architecture. You can choose to implement business interfaces as POJOs (Plain Old Java Object) or local EJBs without affecting calling code.
- Spring helps you solve many problems without using EJB. Spring can provide an alternative to EJB that's appropriate for many applications. For example, Spring can use AOP to deliver declarative transaction management without using an EJB container; even without a JTA implementation, if you only need to work with a single database.
- Spring provides a consistent framework for data access, whether using JDBC or an O/R mapping product such as TopLink, Hibernate or a JDO implementation.
- Spring provides a consistent, simple programming model in many areas, making it an ideal architectural "glue." You can see this consistency in the Spring approach to JDBC, JMS, JavaMail, JNDI (Java Naming and Directory Interface) and many other important APIs.
Spring is a powerful framework that solves many common problems in J2EE (Java 2 Platform, Enterprise Edition). Many Spring features are also usable in a wide range of Java environments, beyond classic J2EE.
Spring provides a consistent way of managing business objects and encourages good practices such as programming to interfaces, rather than classes. The architectural basis of Spring is an Inversion of Control container based around the use of JavaBean properties. However, this is only part of the overall picture: Spring is unique in that it uses its IoC container as the basic building block in a comprehensive solution that addresses all architectural tiers.
Spring provides a unique data access abstraction, including a simple and productive JDBC framework that greatly improves productivity and reduces the likelihood of errors. Spring's data access architecture also integrates with TopLink, Hibernate, JDO and other O/R mapping solutions.
Spring also provides a unique transaction management abstraction, which enables a consistent programming model over a variety of underlying transaction technologies, such as JTA (Java Transaction API) or JDBC (JDBC is a Java-based data access technology (Java Standard Edition platform) from Sun Microsystems, Inc.. It is not an acronym as it is unofficially referred to as Java Database Connectivity).
Spring provides an AOP framework written in standard Java, which provides declarative transaction management and other enterprise services to be applied to POJOs or - if you wish - the ability to implement your own custom aspects. This framework is powerful enough to enable many applications to dispense with the complexity of EJB, while enjoying key services traditionally associated with EJB.
Spring also provides a powerful and flexible MVC (Model-View-Controller) web framework that is integrated into the overall IoC container.
source : ibm
Great post.
ReplyDeletehttps://www.producthunt.com/@james_larue